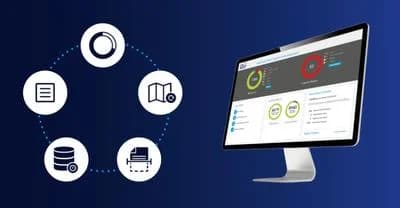
Linux Project: A Cool System Monitoring Tool
Raymond Nwambuonwo / October 8, 2024
Linux Project: A Cool System Monitoring Tool
Table of Contents
What is this tool?
Imagine you have a special pair of glasses that let you see inside your computer. That's kind of what this tool does! It's like a friendly robot that peeks inside your computer and tells you what's going on. And as always, I like explaining things in a digestible way for easier understanding.
What does it do?
Our tool does four main things:
- It checks how hard your computer's brain (CPU) is working.
- It sees how much of your computer's memory is being used.
- It looks at how full your computer's storage is.
- It makes a list of all the programs that are running right now.
Then, it shows you all this information and updates it every 5 seconds.
How I made it
To make this tool, I followed these steps:
- First, I learned about a special helper called "psutil". It's a component that can easily look inside the computer.
- I wrote a set of instructions (referred to as a "script") using a language called Python. Python is like giving instructions to a friendly dragon - it's powerful but easy to talk to!
- I told the dragon (Python) to use the superhero (psutil) to look at different parts of the computer.
- I made the dragon repeat these steps over and over, so we always have new information.
How to use it
Using this tool is pretty easy! Just follow these steps:
- Make sure you have Python on your computer.
- Install the helper. Type this in your computer's command center:
pip install psutil
- Save our instructions in a file called
system_monitor.py
. - Tell the computer it's okay to run our file:
chmod +x system_monitor.py
- Run the file by typing:
python3 system_monitor.py
- Watch as the information appears and updates every 5 seconds!
Understanding the code
Let's look at our instructions and see what each part does:
import psutil
import time
These lines are like telling our dragon to bring two helpers: psutil (our superhero) and time (which helps us wait between updates).
def get_cpu_usage():
return psutil.cpu_percent(interval=1)
This part asks our superhero to check how hard the computer's brain is working.
def get_memory_usage():
memory = psutil.virtual_memory()
return memory.percent
Here, we're asking about the computer's memory - like how many things it's trying to remember at once.
def get_disk_usage():
disk = psutil.disk_usage('/')
return disk.percent
This checks how full the computer's storage box is.
def get_running_processes():
processes = []
for proc in psutil.process_iter(['pid', 'name', 'status']):
if proc.info['status'] == psutil.STATUS_RUNNING:
processes.append(proc.info)
return processes
This part makes a list of all the programs that are awake and doing things right now.
def main():
while True:
print("\n--- System Monitor ---")
print(f"CPU Usage: {get_cpu_usage()}%")
print(f"Memory Usage: {get_memory_usage()}%")
print(f"Disk Usage: {get_disk_usage()}%")
print("\nRunning Processes:")
for proc in get_running_processes():
print(f"PID: {proc['pid']}, Name: {proc['name']}")
time.sleep(5) # Wait for 5 seconds before the next update
This is the main part of our tool. It:
- Prints a title
- Shows how busy the CPU, memory, and storage are
- Lists all the running programs
- Waits for 5 seconds
- Then does it all over again!
if __name__ == "__main__":
main()
This last part is like saying "Okay, dragon, start doing all these things now!"
The line if __name__ == "__main__":
is a common Python idiom. Here's what it
means:
-
__name__
is a special variable that Python creates for each script. It's like a name tag for the script. -
When you run a Python script directly, Python sets
__name__
to the string"__main__"
for that script. -
If the script is imported as a module into another script,
__name__
is set to the name of the script/module instead.
So, this line is basically asking: "Is this script being run directly (not being imported)?"
- If yes (i.e.,
__name__
is"__main__"
), then run themain()
function. - If no (i.e., the script is being imported into another script), don't run
main()
.
This is useful because:
- It allows you to write code that can be either run directly or imported as a module.
- When you import this script into another script, the
main()
function won't automatically run.
Here's a simple analogy:
Imagine you have a toy robot. The robot has a special button that makes it
dance. The line if __name__ == "__main__":
is like asking, "Is someone playing
with me directly?" If yes, the robot dances (runs the main()
function). If the
robot is just sitting on a shelf (being imported but not used directly), it
doesn't dance.
In our system monitoring tool, this line ensures that the monitoring only starts when you run the script directly, not if you were to import its functions into another script.
And that's it! Now you know how our cool system monitoring tool works. It's like having X-ray vision for your computer!